跨站点共享Session解决方案、单点登录解决方案(.NET 2.0版本)
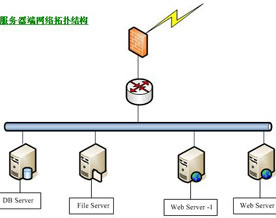
3、创建一个序列化、反序列化操作的类:ShareSessionFormatter
代码如下:
using System;
using System.Web;
using System.Data;
using System.Collections.Generic;
using System.Text;
using System.Runtime.Serialization.Formatters.Soap;
using System.Runtime.Serialization.Formatters.Binary;
using System.Collections;
using System.IO;
namespace ShareSession
...{
/**//* *****************************
*
* Author : Xiaojun Liu
* Create Date : 2007.11.09
* Description :
*
* *****************************
*/
/**//// <summary>
/// 格式化操作器
/// </summary>
public class ShareSessionFormatter
...{
private static string _ShareSessionPath = System.Configuration.ConfigurationManager.AppSettings["ShareSessionPath"].ToString();
/**//// <summary>
/// 序列化格式器类型
/// </summary>
public enum FormatterType
...{
Binary,
Soap
};
/**//// <summary>
/// 执行序列化
/// </summary>
/// <param name="formatterType">类型</param>
public static void Serialize(FormatterType formatterType)
...{
if (HttpContext.Current.Session["AUTH_GUID"] == null)
...{
HttpContext.Current.Session["AUTH_GUID"] = Guid.NewGuid().ToString();
}
Hashtable ht = new Hashtable();
//遍历Session
foreach (string key in HttpContext.Current.Session.Contents.Keys)
...{
ht.Add(key, HttpContext.Current.Session[key]);
}
/**/////执行序列化
switch (formatterType)
...{
case FormatterType.Binary:
BinarySerialize(ht);
break;
case FormatterType.Soap:
SoapSerialize(ht);
break;
default:
break;
}
}
/**//// <summary>
/// 按照Binary格式序列化
/// </summary>
/// <param name="ht"></param>
private static void BinarySerialize(Hashtable ht)
...{
string guid = HttpContext.Current.Session["AUTH_GUID"].ToString();
SessionEntity obj = new SessionEntity(guid);
obj.HtShareSession = ht;
// 创建一个文件guid.xml
Stream stream = File.Open(_ShareSessionPath + guid + ".xml", FileMode.Create);
BinaryFormatter formatter = new BinaryFormatter();
//执行序列化
formatter.Serialize(stream, obj);
stream.Close();
// 将对象置空
obj = null;
}
/**//// <summary>
/// 按照Soap格式序列化
/// </summary>
/// <param name="ht"></param>
private static void SoapSerialize(Hashtable ht)
...{
string guid = HttpContext.Current.Session["AUTH_GUID"].ToString();
SessionEntity obj = new SessionEntity(guid);
obj.HtShareSession = ht;
// 创建一个文件guid.xml
Stream stream = File.Open(_ShareSessionPath + guid + ".xml", FileMode.Create);
SoapFormatter formatter = new SoapFormatter();
//执行序列化
formatter.Serialize(stream, obj);
stream.Close();
// 将对象置空
obj = null;
}
/**//// <summary>
/// 反序列化
/// </summary>
/// <param name="formatterType">传送端的Session["AUTH_GUID"]</param>
/// <param name="guid"></param>
public static void Deserialize(FormatterType formatterType, string guid)
...{
switch (formatterType)
...{
case FormatterType.Binary:
BinaryDeserialize(guid);
break;
case FormatterType.Soap:
SoapDeserialize(guid);
break;
default:
break;
}
}
/**//// <summary>
/// 以Binary方式反序列化
/// </summary>
private static void BinaryDeserialize(string guid)
...{
SessionEntity obj = new SessionEntity(guid);
// 打开文件guid.xml
Stream stream = File.Open(_ShareSessionPath + guid + ".xml", FileMode.Open);
BinaryFormatter formatter = new BinaryFormatter();
obj = (SessionEntity)formatter.Deserialize(stream);
stream.Close();
Hashtable ht = obj.HtShareSession;
obj = null;
//遍历ht,生成Session
CreateSession(ht);
}
/**//// <summary>
/// 以Soap方式反序列化
/// </summary>
private static void SoapDeserialize(string guid)
...{
SessionEntity obj = new SessionEntity(guid);
// 打开文件guid.xml
Stream stream = File.Open(_ShareSessionPath + guid + ".xml", FileMode.Open);
SoapFormatter formatter = new SoapFormatter();
obj = (SessionEntity)formatter.Deserialize(stream);
stream.Close();
Hashtable ht = obj.HtShareSession;
obj = null;
//遍历ht,生成Session
CreateSession(ht);
}
/**//// <summary>
/// 遍历Hashtable,同时创建Session
/// </summary>
/// <param name="ht"></param>
private static void CreateSession(Hashtable ht)
...{
foreach (DictionaryEntry de in ht)
...{
HttpContext.Current.Session[de.Key.ToString()] = de.Value;
}
}
}//
}//